How to Do Email Validation in Python in 5 Easy Steps
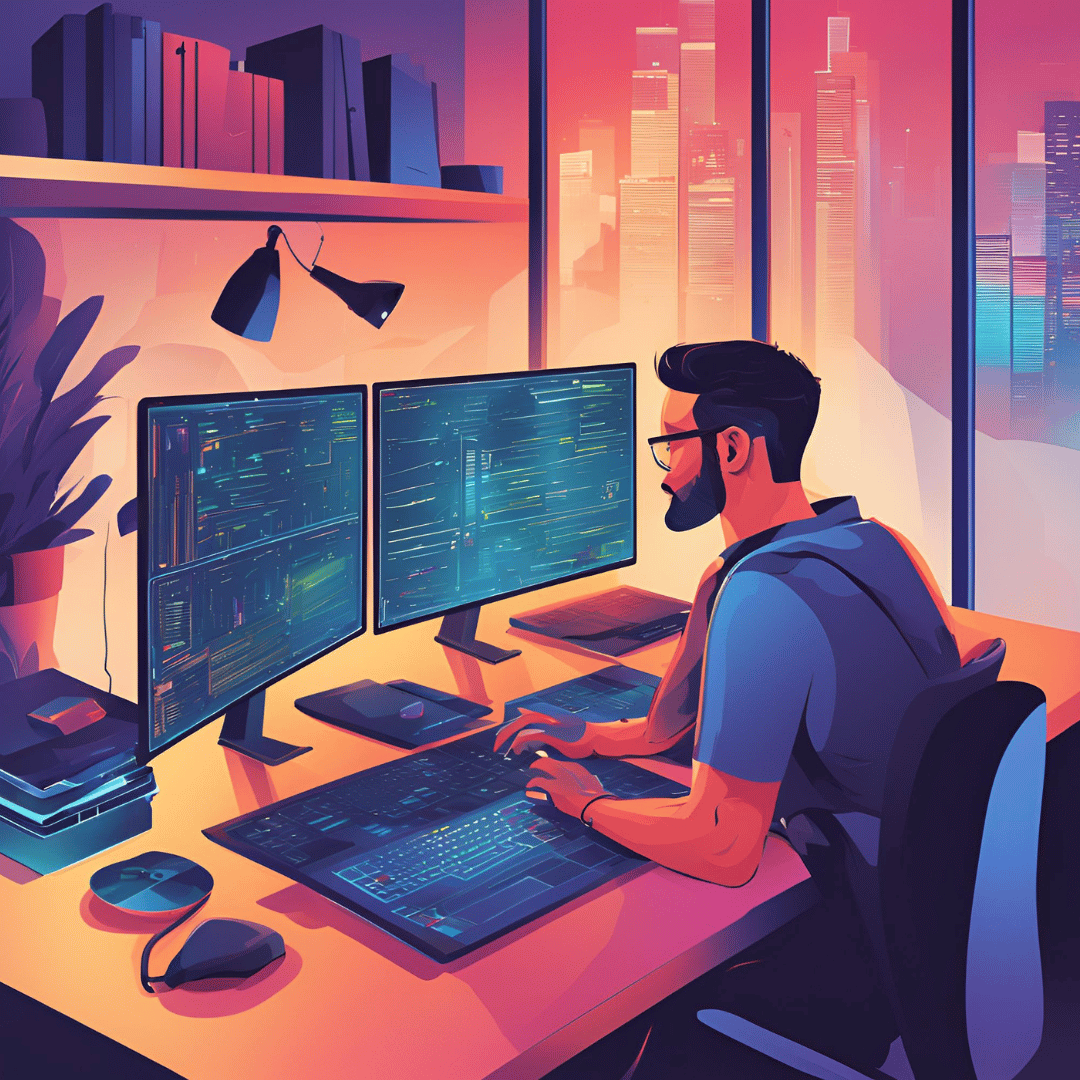
Email verification is essential for maintaining a clean email list and ensuring deliverability. This guide will walk you through verifying email addresses using Python, including checking for open ports, understanding SMTP ports, avoiding spam, and handling greylisting timeouts.
Step 1: Basic Syntax Validation Start by ensuring the email address is in a valid format using regular expressions:
import re
def is_valid_email(email):
regex = r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$'
return re.match(regex, email) is not None
email = "example@example.com"
print(is_valid_email(email)) # Returns True if valid
Step 2: Check Domain MX Records Next, check if the domain has valid MX (Mail Exchange) records:
import dns.resolver
def has_mx_record(domain):
try:
records = dns.resolver.resolve(domain, 'MX')
return len(records) > 0
except (dns.resolver.NoAnswer, dns.resolver.NXDOMAIN):
return False
domain = "example.com"
print(has_mx_record(domain)) # Returns True if MX records exist
Step 3: Check for Open SMTP Ports Before performing an SMTP check, verify that the email server's SMTP ports are open. Common SMTP ports include:
- Port 25: Default SMTP port.
- Port 465: SMTP over SSL.
- Port 587: SMTP with STARTTLS (recommended).
Check if these ports are open:
import socket
def is_port_open(domain, port):
try:
with socket.create_connection((domain, port), timeout=10):
return True
except (socket.timeout, socket.error):
return False
smtp_ports = [25, 465, 587]
domain = "example.com"
for port in smtp_ports:
print(f"Port {port}: {'Open' if is_port_open(domain, port) else 'Closed'}")
Step 4: Handling Greylisting Timeouts Greylisting is an anti-spam technique where a mail server temporarily rejects an email from an unknown sender, asking them to retry later. To handle greylisting:
- Retry After Delay: If you receive a 450 SMTP error code, wait and try sending the email again after a few minutes.
Here’s how you can simulate this in your script:
import time
def verify_email_smtp(email, port=587):
domain = email.split('@')[1]
try:
records = dns.resolver.resolve(domain, 'MX')
mx_record = records[0].exchange.to_text()
server = smtplib.SMTP(mx_record, port)
server.set_debuglevel(0)
server.helo()
server.mail('youremail@example.com')
code, message = server.rcpt(email)
server.quit()
if code == 450: # Greylisting detected
print("Greylisting detected, retrying after delay...")
time.sleep(60) # Wait for a minute before retrying
return verify_email_smtp(email, port)
return code == 250 # Valid email if code 250
except Exception as e:
return False
email = "example@example.com"
print(verify_email_smtp(email)) # Returns True if the email is valid
Step 5: Avoiding Spam Avoid behaviors that can trigger spam filters:
- Limit Verification Attempts: Avoid making too many requests in a short period.
- Use a Real Sending Email: Use a real and active email address in the
MAIL FROM
command. - Check for Catch-All Domains: Some domains accept all emails, which might give false positives.
Summary of Steps:
- Syntax Validation: Ensure email format is correct.
- MX Record Check: Verify the domain has an active mail server.
- Port Check: Ensure SMTP ports are open (25, 465, 587).
- SMTP Simulation & Greylisting: Perform a non-intrusive check and handle greylisting.
- Avoid Spam: Follow best practices to avoid spam traps.
Python Email Verification Guide with Tomba.io API
Email verification is a crucial step to ensure your emails reach their intended recipients. Using Tomba.io's API with Python, you can easily verify the validity of any professional email address. Here's how you can do it:
Step 1: Install the Tomba Python Client First, install the Tomba Python package if you haven't already:
pip install tomba-io
Step 2: Initialize the Tomba Client Set up the client with your API key and secret:
from tomba.client import Client
from tomba.services.verifier import Verifier
client = Client()
client.set_key('your_api_key').set_secret('your_secret_key')
Verify the deliverability of any professional email address:
from tomba.client import Client
from tomba.services.verifier import Verifier
client = Client()
client.set_key('your_api_key').set_secret('your_secret_key')
verifier = Verifier(client)
result = verifier.email_verifier('b.mohamed@tomba.io')
print(result)
🔗 Get Started with Tomba: Sign up for a free account at Tomba.io. The free plan includes 25 search requests and 50 verifications per month. For more volume and features, check out the pricing plans.
Integrate Tomba into your workflow and supercharge your email discovery and verification process with Python today!
By incorporating these steps into your Python scripts, you can effectively verify email addresses while handling greylisting and minimizing the risk of triggering spam defenses.